It seems that there is a correct sequence for creating a Response
object with a set-cookie header and a JSON body in Next.js 13.
I’m not sure if this is limited to the beta app directory version and typescript.
Problem: typescript is confused?
If you set the cookie first and then try to use the Next.Response
JSON method to create a JSON body, typescript yells at you and says “expected 0 arguments, but got 2”.
This is because it is getting confused with the json()
method from the Response web API.
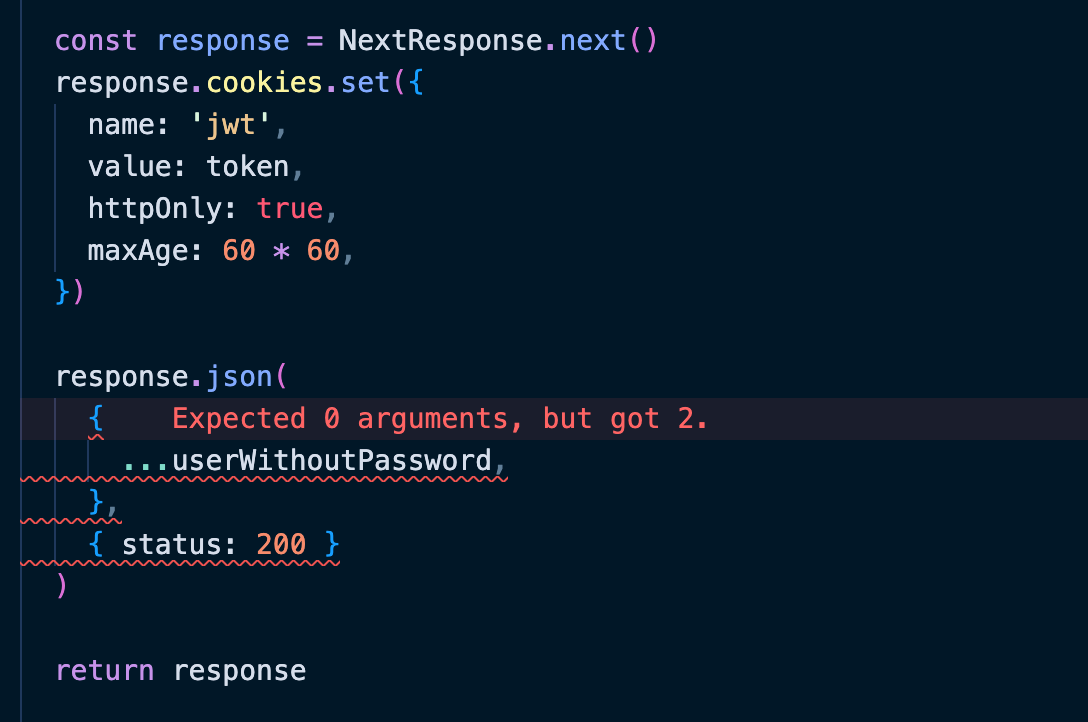
Solution: create a response with JSON body first then set the cookie
So the simple solution is to create your NextResponse.json()
first then set the cookie.
import { NextRequest, NextResponse } from 'next/server'
export async function POST(request: NextRequest) {
// ...your post request logic here
// Set json response first
const response = NextResponse.json(
{
...userWithoutPassword,
},
{ status: 200 }
)
// Then set a cookie
response.cookies.set({
name: 'jwt',
value: token,
httpOnly: true,
maxAge: 60 * 60,
})
return response
}
Hope this was useful!
Leave a Reply